Python 基础练习题
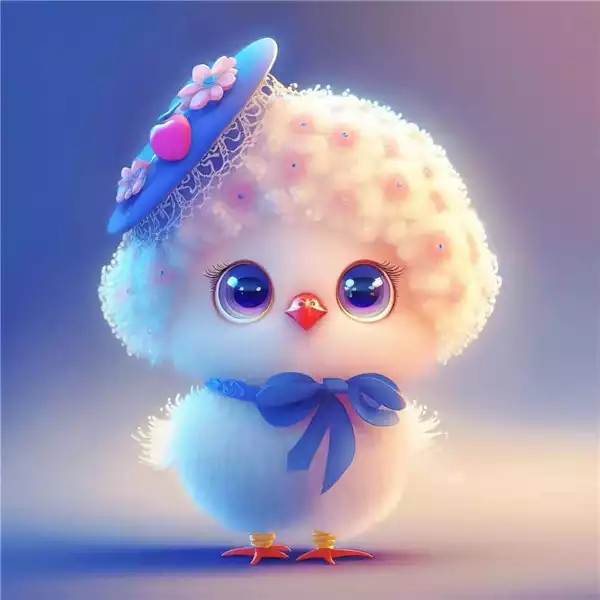
AI-摘要
Tianli GPT
AI初始化中...
介绍自己 🙈
生成本文简介 👋
推荐相关文章 📖
前往主页 🏠
前往爱发电购买
Python 基础练习题
Pupper练习题 1
题目要求
有一个数据list of dict如下
a = [{“test1”: “123456”},{“test2”: “123456”},{“test3”: “123456”}]
写入到本地一个txt文件,内容格式如下:
test1,123456
test2,123456
test3,123456
案例源码
1 | a = [{"test1": "123456"},{"test2": "123456"},{"test3": "123456"}] |
练习题 2
题目要求
a = [1, 2, 3, 4, 5]
b = [“a”, “b”, “c”, “d”, “e”]
如何得出c = [“a1”, “b2”, “c3”, “d4”, “e5”]
案例源码
1 | a = [1, 2, 3, 4, 5] |
练习题 3
题目要求
写一个小程序:控制台输入邮箱地址(格式为 username@companyname.com), 程序识别用户名和公司名后,将用户名和公司名输出到控制台。
要求:
- 校验输入内容是否符合规范(xx@polo.com), 如是进入下一步,如否则抛出提 示”incorrect email format”。注意必须以.com 结尾
- 可以循环“输入—输出判断结果”这整个过程
- 按字母 Q(不区分大小写)退出循环,结束程序
案例源码
1 | while True: |
练习题 4
题目要求
如果一个 3 位数等于其各位数字的立方和,则称这个数为水仙花数。
例如:153 = 1^3 + 5^3 + 3^3,因此 153 就是一个水仙花数
那么问题来了,求1000以内的水仙花数(3位数)
案例源码
1 | lists = [] |
评论
匿名评论隐私政策
✅ 你无需删除空行,直接评论以获取最佳展示效果